Deck Of Cards

60 Card Deck - WHITE ANGELS - Ready to Play - Fun - Magic the Gathering MTG Atratis1978. 5 out of 5 stars (245) $ 25.99 FREE shipping Only 1. “Deck of Cards” was written in 1948 by T. Texas Tyler, who also released it as a single. Tex Ritter covered it that same year and it was a top ten hit on the Billboard Country charts.
There's something quite enchanting about finding the right set of tarot cards to help you divine your past, present and future. Even so, an actual 78-card tarot deck isn't completely essential if you're curious about conducting readings.
In fact, if you know what you're doing, you can do tarot readings with a regular old 52-playing card deck. Keep reading to find out how it's done.
Cleanse Your Cards
Regardless of whether you're using a brand new deck from the shop or an old pack of cards you find lying around the house, you'll want to cleanse and activate them before you start your readings. It's said that this is because they may have been exposed to other people's energies of the past, and you'll want to dispel them to protect yourself and the person being read.
There are a number of methods for cleansing your cards, but we recommend beginning by sorting the cards, Aces to Kings, in whatever suit order suits your fancy. Not only will this bestow your energy upon the cards, but it'll help you discover any missing ones! Once that process is completed, shuffle them back up. From there, you can burn sage and run the cards through the smoke, leave the deck on the window sill during a full moon, or simply leave a healing crystal on top of the deck overnight.
Once that process is completed, you'll also want to keep your cards in a private space, where they won't be exposed to other people's energies. Also do a new cleansing anytime someone else touches the cards, or when you feel the readings have been particularly negative or unclear. It's possible that all they need is a refresh.
(via Unsplash)
Learn the Suits
Learning how to read a standard deck of playing cards as if they were playing cards begins with knowing how the suits translate. Hearts can be read as Cups, Spades as Swords, Diamonds as Pentacles and Clubs as Wands. Learning how the cards correlate can also help you master the meanings of the tarot cards more quickly without having to look them up every time. Hearts (Cups) have to do with emotions and matters of the heart, while Spades (Swords) regard thought and communication. Diamonds (Pentacles) are related to possessions and money, while Clubs (Wands) correlate with movement and imagination.
Learn the Court Cards
Learning what the court cards represent is simpler because there's a more direct correlation here between playing cards and tarot cards. Aces are aces, twos are twos, and so on. The only difference between the playing card deck and the Minor Arcana is that playing cards lack the Page cards, and that the playing card Jacks translate to tarot Knights.
If your deck includes Jokers, you can also keep one inside your deck to represent the Fool. While it might not sound that positive, this card represents new beginnings and having faith in the universe, so it can be a valuable addition to your deck.

(via Unsplash)
Have a Reference Guide
If you're new to tarot readings, the thought of reading your own cards can be quite intimidating. Lucky for both new and experienced tarot readers, there are tons of handy references out in the world to explore. Maybe you'll want to use the internet as your guide, or invest in an approachable tarot book. Personally, we love The Only Tarot Book You'll Ever Needand Tarot for Self-Care, but don't be afraid to head out to the library or a bookstore to find the perfect fit for you.
Understand the Limitations
While a regular pack of playing cards can stand in as an excellent substitute for a traditional tarot deck, it's also important to understand that a playing card reading has some limitations. While most tarot decks include 78 cards, a playing card deck only has 52 (53, if you're counting the Joker). Missing the Major Arcana (as well as each suit's Page cards) means having limited options, which might result in a reading that isn't as in-depth as possible, or doesn't provide the insight that might otherwise be available. We recommend doing smaller spreads or single-card readings using this method, rather than large ones with four cards are more, for the best results.
(via Unsplash)
Need the perfect tarot reference book? Click HERE to find out our favorite things we learned from The Only Tarot Book You'll Ever Need.
Description:
Published: May 14, 2020 favorite0 forum0 poll346
By: Javier Hernandez, Florida International University
Category: Computer Science
Hashtags: #Coding#JAVA
ArrayLists
I. The Assignment
Card players typically like to keep the cards in their hand sorted by suit and within each suit by face value. Assuming that a hand consists of 13 cards, write a Java program to insert each card into an initially empty hand in the proper order, so that there is no need to sort the hand.
The order of the suits is unimportant but within each suit the order of the cards should be 2, 3, 4, 5, 6, 7, 8, 9, 10, J, Q, K, A (i.e. the Ace is always the high card).
II. The Card Class
Begin by creating a class to model a playing card. The Card class will have two private instance variables: (1) the face value (aka: “rank”) stored as ints 2..14 and (2) the suit (Spades, Hearts, Diamonds, Clubs).
Your Card class will have these methods:
1. a proper constructor that creates a Card with a given rank and suit
2. an accessor (“get”) method that returns the rank
3. an accessor (“get”) method that returns the suit
4. a toString method that returns a String representation of a card as shown in these examples: A♠, 10♣, 7♦, Q♥
To get the symbols for the suits, use the escape sequences for the Unicode characters:
“u2660” (Spade) “u2663” (Club)
“u2665” (Heart) “u2666” (Diamond)
III. The Deck Class
Create a class to model a standard 52-card deck. Your Deck class will have a private instance variable that is an ArrayList-of-Card. (You may have additional instance var’s although none are needed)
A Deck Of Playing Cards
Your Deck class will have these methods:
1. a proper constructor that creates a standard Deck of 52 cards
2. a method that deals the top card. I.e. returns the Card object on top of the Deck
3. a method that shuffles the deck. To get credit for this method you must use this algorithm:
for each card in the deck
exchange it with the card at a randomly-selected index
(to “mix ‘em up good” you might want to shuffle the deck a number of times)
IV. The Hand Class
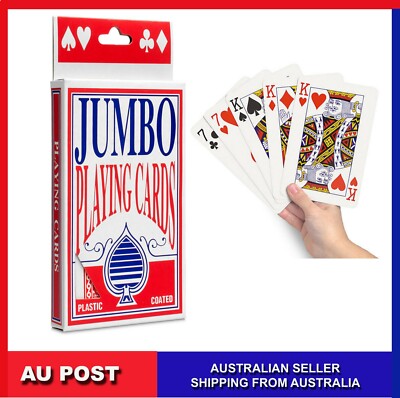
Create a class to model a hand of 13 Cards. Your Hand class will have a private instance variable that is an ArrayList-of-Card, and these methods:
1. a proper constructor that creates an empty Hand
2. a method to fill a Hand with 13 Cards dealt from a Deck, with each one being inserted in its proper place in the Hand
3. a toString method that returns a String representation of the Hand (Hint: call the Card class toString method for each Card in the Hand)
V. The Test Class
Your test class will have a main method that creates a Hand, calls the method that fills it, calls the toString method for the Hand, and prints the String returned. After each Hand is displayed, ask the user if she wants to see another.
VI. Specifications
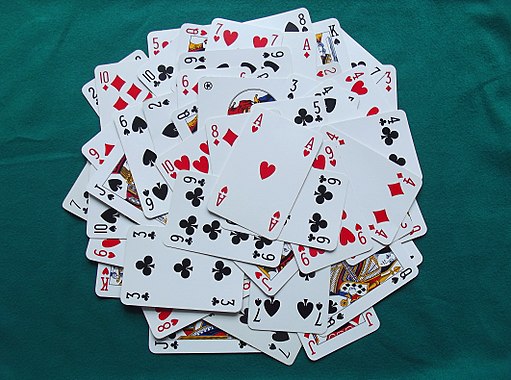
1. You must use a generic “ArrayList of Card” as the implementation of the Deck and the Hand
No credit will be given if any other data structures, including arrays, are used anywhere in the program
2. No credit for using any of the methods of Java’s Collections class (e.g. shuffle). Use the shuffle algorithm given above
3. Although the assignment calls for a Hand of 13 cards and a Deck of 52, you should “parameterize” your program by using defined constants for the number of cards in the Hand and in the Deck. That way, you can generate hands and decks of different sizes by changing only the constant declarations
4. As specified above, none of the methods of your Deck, Hand, and Card classes do any output. All output is to be done in main
Make sure your Deck, Hand, and Card classes all contain proper Java “documentation comments” and adhere to all the style and internal documentation standards discussed in class and covered in Unit 1
VII. Upload 2 Files to Canvas
1. A zip file of your NetBeans project folder
2. A Word doc with your name to receive feedback
Do not zip the individual java files; zip the project folder. Include a file containing the output of 3 hands. See the “Using NetBeans” doc, part XI for an easy way to include the output in the project
VIII. Due Date – Tuesday, January 28th
You will receive two separate grades for this assignment – one for the program itself and one for the web pages created by Javadoc
To make sure you receive credit, review the “Submitting Your Assignments” and “Creating a Zip File” docs in the “Before Beginning” Unit online
Do not zip the project from within NetBeans! If you do, the zip will not contain the dist folder (where the html files are stored) and you will be unhappy with the grade
Algorithm to Create the Sorted Hand
Place the first card in the hand
For each additional card, do the following:
Get the suit
If the card is of a suit that is not yet in the Hand
Insert it as the new last card in the Hand
Else, do the following:
If the value is higher than all the cards of that suit
Insert it as the new last card of that suit

Else
Insert it just before the first card of that suit with a higher value
Optional Easier Algorithm to Create the Sorted Hand
Create an ArrayList of Cards for each suit
Large Printable Deck Of Cards
For each card, insert it in its proper place in the list for that suit, using the techniques described above
Add each suit list to the Hand
Hopefully Helpful Hints
1. Begin by creating all classes with method “stubs” only. A method stub is just the Javadoc comment, the method heading, and an empty body. If the method returns a value, use some temporary return value. This way, the entire program “skeleton” will compile and execute and you can fill in each method as you figure it out
2. Since the Hand class method fill is the most challenging, start with a temporary version that simply adds each card to the hand.
That way, you can test and verify that all the other methods are correct – and have the majority of the credit “in the bank” – before turning your full attention to the full-blown fill
Personalized Deck Of Cards
Standard Deck Of Cards
3. Hint: To enable your Hand class to seamlessly access the Deck, create a Deck object in your Hand cla
Attachments:
Deck Of Cards Workout
File | TheCard.txt | 2.0 KB |
File | TheDeck.txt | 4.4 KB |
File | TheHand.txt | 4.5 KB |
File | TheTest.txt | 1.4 KB |